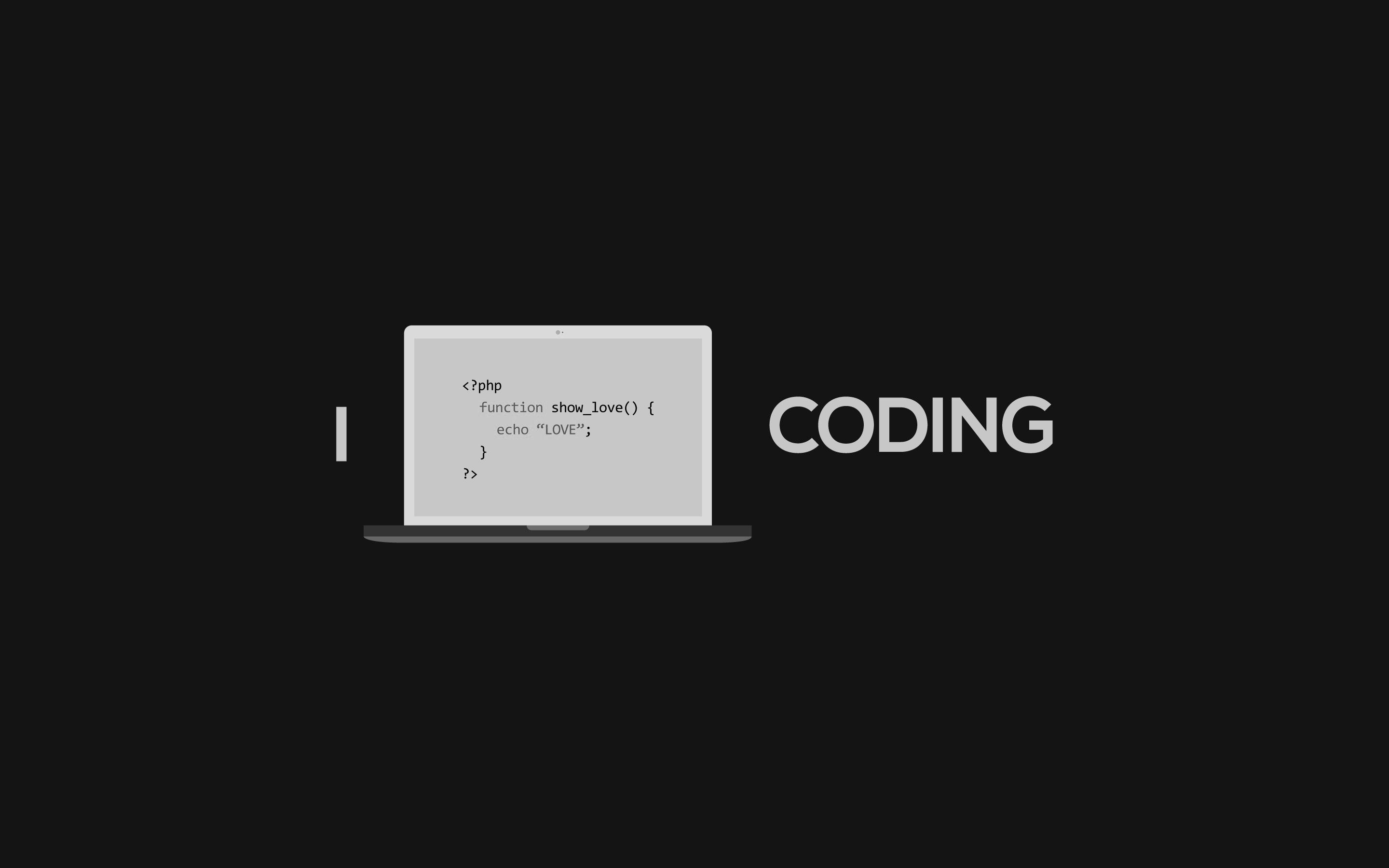
Casos de Programación en PHP con MySQL Consultas Básicas
En esta ocasión realizaremos varios casos en PHP donde la aplicación pueda realizar consultas hacia la base de datos, extraer datos y mostrarlos en la aplicación web.
Crear la base de datos PRACTICA1 con la tabla CLIENTES con los campos (código, nombre, apellidoPaterno, apellidoMaterno, telefono), ingresar 10 clientes, programar una página web que permita visualizar todos los clientes y que permita agregar nuevos clientes.
Creando la base de datos CREATE DATABASE practica1; CREATE TABLE clientes ( codigo varchar(50) NOT NULL, nombre varchar(50) NOT NULL, apellidoPaterno varchar(50) NOT NULL, apellidoMaterno varchar(50) NOT NULL, telefono varchar(15) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4; INSERT INTO `clientes` VALUES('CLI001', 'JUAN', 'PEREZ', 'MEND', '2241542'); INSERT INTO `clientes` VALUES('CLI002', 'MARIA', 'MENDOZA', 'JIMENEZ', '564656'); INSERT INTO `clientes` VALUES('CLI003', 'ROBERTO', 'GOMEZ', 'MENDOZA', '564556'); INSERT INTO `clientes` VALUES('CLI004', 'MANUEL', 'FERNANDEZ', 'GARCIA', '524252'); INSERT INTO `clientes` VALUES('CLI005', 'ANDREA', 'PRIETO', 'ARONES', '454656'); INSERT INTO `clientes` VALUES('CLI006', 'JOSE', 'JIMENEZ', 'MENDIOLA', '456531'); INSERT INTO `clientes` VALUES('CLI007', 'HUMBERTO', 'JUAREZ', 'REGLIATI', '455633'); INSERT INTO `clientes` VALUES('CLI008', 'VICTORIA', 'HERNANDEZ', 'HERNANDEZ', '654655'); INSERT INTO `clientes` VALUES('CLI009', 'HILDA', 'GUTIERREZ', 'SANDION', '455664'); INSERT INTO `clientes` VALUES('CLI010', 'MARTIN', 'ESCALANTE', 'MENDOZA', '456556'); INSERT INTO `clientes` VALUES('CLI011', 'JORGE', 'HERRERA', 'JORSE', '543545');
Una vez creada la base de datos y las tablas correspondientes con sus datos iniciales, crearemos la aplicación en php, de tal forma que permita realizar una consulta simple a la base de datos y mostrarlos en una tabla, el primer caso tratará de obtener los datos de la tabla clientes y mostrarlos.
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Documento sin título</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" integrity="sha384-9aIt2nRpC12Uk9gS9baDl411NQApFmC26EwAOH8WgZl5MYYxFfc+NcPb1dKGj7Sk" crossorigin="anonymous"> </head> <body> <?php $cn=mysqli_connect("localhost","root","","practica1"); if(isset($_POST['Agregar'])) { if ($_POST['Agregar']=='Agregar') { $xcod=$_POST['Text1']; $xnombre=$_POST['Text2']; $xapePaterno=$_POST['Text3']; $xapeMaterno=$_POST['Text4']; $xtelefono=$_POST['Text5']; $consulta="insert into clientes values ('$xcod','$xnombre','$xapePaterno','$xapeMaterno','$xtelefono')"; if (mysqli_query($cn,$consulta)) { echo "Registro Exitoso"; } } } ?> <form id="form1" name="form1" method="post" action=""> <table class="table table-responsive table-striped table-hover table-borderless table-sm" width="200" border="1"> <tr class="thead-dark"> <td colspan="6">CLIENTES</td> </tr> <tr> <td>CODIGO</td> <td>NOMBRE</td> <td>APELLIDOS PATERNO</td> <td>APELLIDOS MATERNO</td> <td>TELEFONO</td> <td> </td> </tr> <tr> <td><input type="text" name="Text1" id="Text1" /></td> <td><input type="text" name="Text2" id="Text2" /></td> <td><input type="text" name="Text3" id="Text3" /></td> <td><input type="text" name="Text4" id="Text4" /></td> <td><input type="text" name="Text5" id="Text5" /></td> <td><input type="submit" name="Agregar" id="Agregar" value="Agregar" /></td> </tr> <?php $c=0; $rs=mysqli_query($cn,"select * from clientes"); while($cabecera=mysqli_fetch_field($rs)) { $campos[$c]=$cabecera->name; $c++; } while ($resultado=mysqli_fetch_array($rs)) { echo "<tr>"; foreach($campos as $colum) { echo "<td>", $resultado[$colum]," </td>"; } echo "</tr>"; } ?> </table> </form> </body> </html>
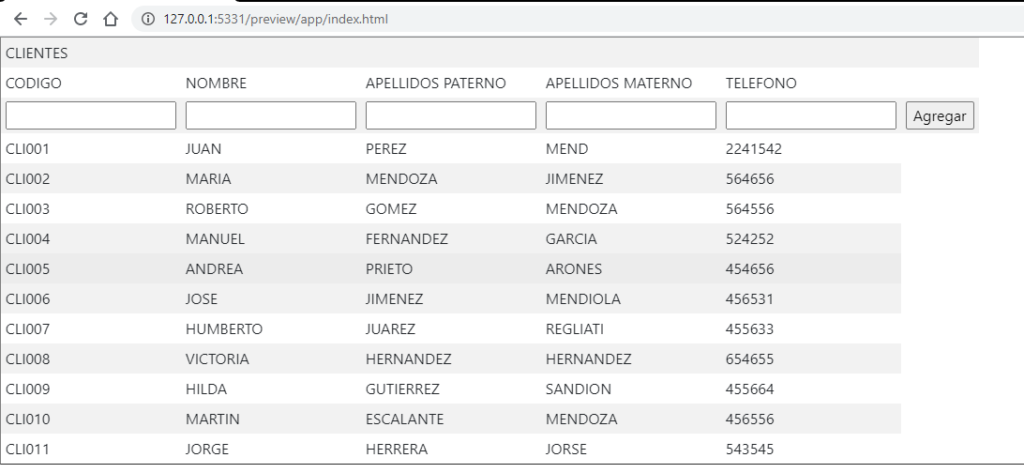
En la base de datos PRACTICA1 crear una tabla PRODUCTOS con los campos (código, nombre, precio y stock) , ingresar 10 producto, programar una página web que permita visualizar todos los productos y que permita agregar nuevos productos.
CREATE TABLE productos ( codigo varchar(50) NOT NULL PRIMARY KEY, nombre varchar(80) NOT NULL, precio decimal(10,2) NOT NULL, stock int(11) NOT NULL ); INSERT INTO `productos` VALUES('PROD001', 'ARROZ TAILANDEZ', '4.00', 20); INSERT INTO `productos` VALUES('PROD002', 'LECHE GLORIA', '4.00', 20); INSERT INTO `productos` VALUES('PROD003', 'LEJIA SAPOLIO', '5.00', 12); INSERT INTO `productos` VALUES('PROD004', 'AVENA QUAKER', '2.00', 15); INSERT INTO `productos` VALUES('PROD005', 'JABON BOLIVAR', '3.00', 15); INSERT INTO `productos` VALUES('PROD006', 'DETERGENTE ACE', '4.00', 15); INSERT INTO `productos` VALUES('PROD007', 'DETERGENTE BOLIVAR', '4.00', 4); INSERT INTO `productos` VALUES('PROD008', 'JABON NEKO', '4.00', 25); INSERT INTO `productos` VALUES('PROD009', 'PASTA DE DIENTES COLGATE', '4.00', 4); INSERT INTO `productos` VALUES('PROD010', 'PASTA DE DIENTES DENTO', '3.00', 56); INSERT INTO `productos` VALUES('PROD011', 'DESODORANTE REXONA', '3.00', 45);
En este caso trataremos de mostrar los datos de la tabla productos en la aplicación php mediante una tabla.
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Documento sin título</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" integrity="sha384-9aIt2nRpC12Uk9gS9baDl411NQApFmC26EwAOH8WgZl5MYYxFfc+NcPb1dKGj7Sk" crossorigin="anonymous"> </head> <body> <?php $cn=mysqli_connect("localhost","root","","practica1"); if(isset($_POST['Agregar'])) { if ($_POST['Agregar']=='Agregar') { $xcod=$_POST['Text1']; $xnombre=$_POST['Text2']; $xprecio=$_POST['Text3']; $xstock=$_POST['Text4']; $consulta="insert into productos values ('$xcod','$xnombre',$xprecio,$xstock)"; if (mysqli_query($cn,$consulta)) { echo "Registro Exitoso"; } } } ?> <form id="form1" name="form1" method="post" action=""> <table class="table table-responsive table-striped table-hover table-borderless table-sm" width="200" border="1"> <tr class="thead-dark"> <td colspan="6">PRODUCTOS</td> </tr> <tr> <td>CODIGO</td> <td>NOMBRE</td> <td>PRECIO</td> <td>STOCK</td> <td> </td> </tr> <tr> <td><input type="text" name="Text1" id="Text1" /></td> <td><input type="text" name="Text2" id="Text2" /></td> <td><input type="text" name="Text3" id="Text3" /></td> <td><input type="text" name="Text4" id="Text4" /></td> <td><input type="submit" name="Agregar" id="Agregar" value="Agregar" /></td> </tr> <?php $c=0; $rs=mysqli_query($cn,"select * from productos"); while($cabecera=mysqli_fetch_field($rs)) { $campos[$c]=$cabecera->name; $c++; } while ($resultado=mysqli_fetch_array($rs)) { echo "<tr>"; foreach($campos as $colum) { echo "<td>", $resultado[$colum]," </td>"; } echo "</tr>"; } ?> </table> </form> </body> </html>
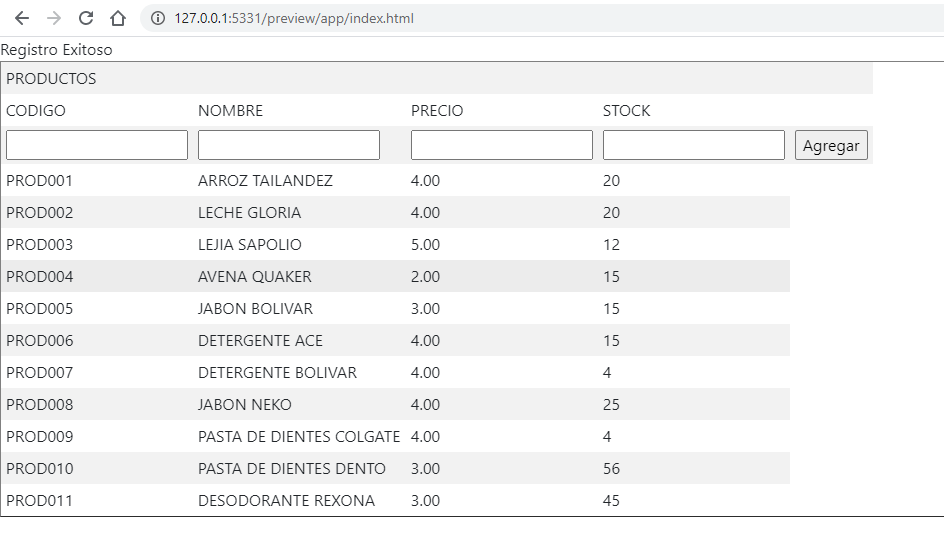
Publicar un comentario
0 Comentarios